Generate an access token
Create an access token to use to authenticate your requests.
Before you can make any requests to the Adfin API, you must generate an access token to authenticate your requests. Adfin uses OAuth 2.0 for secure authentication and authorisation.
Obtain your client credentials
Your Adfin Integration Manager will share encrypted app credentials containing your
client_id
andclient_secret
.
OAuth 2.0 flows supported
- Client credentials flow (for platform-to-platform communication)
- Authorization code flow (for biller authorisation on behalf of your platform)
Client credentials flow
This flow is used when platforms need to access Adfin resources directly.
Request example:
curl -X POST "https://staging.adfin.com/api/oauth2/token" \
-H "Content-Type: application/x-www-form-urlencoded" \
-d '{
"grant_type": "client_credentials",
"client_id": "{your_client_id}",
"client_secret": "{your_client_secret}"
}'
Explanation:
- URL:
https://staging.adfin.com/api/oauth2/token
is the endpoint to request the access token.
- Content-Type:
- The request content type is set to
application/x-www-form-urlencoded
.
- Body:
- The body contains the
grant_type
(set toclient_credentials
) - Replace
your_client_id
andyour_client_secret
with the actual credentials from your Adfin app client.
If successful, Adfin will respond with an access token:
{
"access_token": "your_access_token",
"token_type": "Bearer",
"expires_in": 3600
}
Authorization code flow
This flow allows your platform to request an access token after receiving an authorization code from a biller's login session.
Step 1: Redirect biller to Adfin's authorisation page
Prerequisite
You must share you desired
redirect_uri
with your Adfin Integration Manager. As an additional security measure we will associate this URL with your Adfin app in the backend.
To start, redirect the biller to Adfin’s authorisation page by constructing your unique authorisation url:
https://staging.adfin.com/auth/login?&client_id={your_client_id}&scope=invoices%20customers&redirect_uri={your_redirect_uri}&state={random_state_value}
Explanation:
- client_id: The
client_id
of your platform. - redirect_uri: This is where billers will be sent after granting permission to Adfin (e.g., your homepage). This must match the one configured in Adfin by your Integration Manager.
- scope: Permissions you're requesting. To manage the billers invoices and customers, you need to use the scopes
invoices
andcustomers
. These are currently the only scopes Adfin supports. - state : Optional, but highly recommended parameter that's useful in preventing Cross-site Request Forgery (CSRF) attacks. Provide a randomised state value to Adfin at initiation, and this value will be sent back verbatim appended to the
redirect_uri
after a successful grant. Your application can then verify the returned value.
Here's an example of the Adfin authorisation page that the biller will see once logged in:
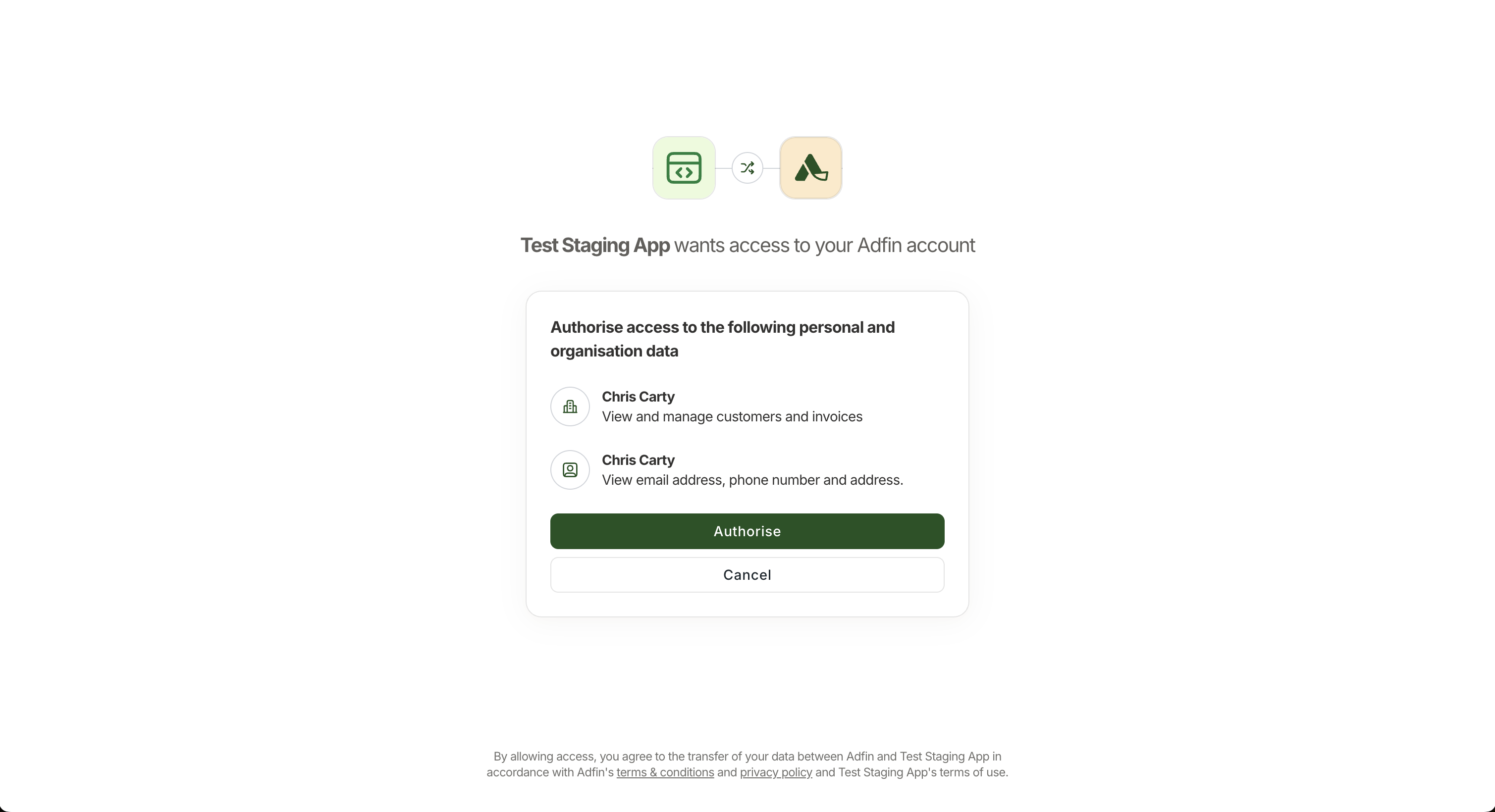
Step 2: Handle the callback
After the biller logs in and approves the authorisation, they will be redirected back to the redirect_uri
configured in Step 1. Adfin will append a code
in the query string, like:
https://your-platform.com/callback?code=[your_authorization_code]&state=random_state_value
Step 3: Exchange the authorization code for tokens
Once you have the authorization code, use this cURL request to exchange it for access and refresh tokens:
curl -X POST "https://staging.adfin.com/api/oauth2/token" \
-H "Content-Type: application/x-www-form-urlencoded" \
-d '{
"grant_type": "authorization_code",
"client_id": "{your_client_id}",
"client_secret": "{your_client_secret}",
"code": "{your_authorization_code}",
"redirect_uri": "{your_redirect_uri}"
}'
Explanation:
- URL:
https://staging.adfin.com/api/oauth2/token
is the endpoint to exchange the authorization code for tokens.
- Headers:
- Set the
Content-Type
header toapplication/x-www-form-urlencoded
.
- Request Body:
grant_type
: Set toauthorization_code
.client_id
: the credentials of your Adfin app.client_secret
: the credentials of your Adfin app.code
: The authorization code you received from the redirect after biller login.redirect_uri
: Must be the same URI used in the initial request.
Step 4: Example response
If successful, Adfin will respond with an access_token
and a refresh_token
:
{
"access_token": "your_access_token",
"refresh_token": "your_refresh_token",
"expires_in": 3600,
"token_type": "Bearer"
}
You can now use the access_token
to make authorised requests on behalf of the biller (e.g Create customers on behalf of the biller).
Using the refresh token
Use the refresh token to request a new access token when the current access token expires. This is critical for maintaining session continuity without requiring the biller to log in again. Below is the process and an example cURL command to guide you.
Step 1: Request a new access token
curl -X POST "https://staging.adfin.com/api/oauth2/token" \
-H "Content-Type: application/x-www-form-urlencoded" \
-d '{
"grant_type": "refresh_token",
"client_id": "{your_client_id}",
"client_secret": "{your_client_secret}",
"refresh_token": "{your_refresh_token}"
}'
Explanation:
- URL:
https://staging.adfin.com/api/oauth2/token
is the endpoint to obtain a new access token using the refresh token.
- Headers:
- Content-Type: Set to
application/x-www-form-urlencoded
.
- Request body:
grant_type
: Set torefresh_token
.client_id
: Your platform'sclient_id
.client_secret
: Your platform'sclient_secret
.refresh_token
: The refresh token you received during the initial token exchange.
Step 2: Example response
If the request is successful, the response will contain a new access token, and optionally, a new refresh token (if it is also being rotated).
{
"access_token": "new_access_token",
"refresh_token": "new_refresh_token",
"expires_in": 3600,
"token_type": "Bearer"
}
Token expiry:
- access_token: Expires after 1 hour.
- refresh_token: Expires after 30 days.
Error handling
- 401 Unauthorized: The refresh token has expired or is invalid. You will will need to prompt the biller to reauthorize via the Authorization Code Flow.
- 400 Bad request: Incorrect request format or missing required parameters.
Best practices for using refresh tokens:
- Token rotation: If the response provides a new refresh token, replace the old one in your system. This helps prevent stale tokens.
- Token storage: Store refresh tokens securely (e.g., encrypted) to protect against token theft.
- Graceful expiry handling: Always handle the expiration of access tokens by using the refresh token to avoid biller login disruptions.
Updated 3 months ago