⚡️ Quickstart: set up a one-time payment
Learn how to prepare for and set up a one-time payment quickly in our staging environment.
Prerequisite
You have obtained a user access token by following the steps in the Authorization flow guide.
Step 1: Create customer
curl -X POST "https://staging.adfin.com/api/customers" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer your_access_token" \
-d '{
"name": "Adfin financial services Ltd",
"people": [
{
"firstName": "John",
"lastName": "Doe",
"email": "[email protected]",
"phoneNo": "+44567123456"
}
],
"addresses": [
{
"city": "London",
"postalCode": "1245",
"country": "UK",
"addressLine1": "Street"
}
]
}'
Explanation:
- URL:
- Use the base URL
https://api.adfin.com/v1/api/customers
for customer creation.
- Use the base URL
- Headers:
Content-Type
: Set toapplication/json
for JSON payloads.Authorization
: Use the Bearer token with your access token for authentication.
- Body:
name
: The name of the customer (e.g.,Adfin financial services Ltd
).people
: An array with the details of associated contacts.addresses
: An array of addresses related to the customer.\
Replace your_access_token
with a valid token for this request.
Example response
{
"id": "066b7125-cc80-40f1-a220-3ef297b979db",
"name": "Adfin financial services Ltd",
"people": [
{
"firstName": "John",
"lastName": "Doe",
"email": "[email protected]",
"phoneNo": "+447123456789"
}
],
"addresses": [
{
"city": "London",
"postalCode": "1245",
"country": "UK",
"addressLine1": "Street"
}
],
"directDebitMandate": {},
"timezone": "Europe/London"
}
Step 2: Create an invoice
Using the id
generated for the customer in Step 1, you can now create an invoice for this customer.
curl -X POST "https://staging.adfin.com/api/invoices" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer your_access_token" \
-d '{
"invoiceNo": "INV-6666",
"customer": {
"customerId": "{customerId}",
"externalCustomerId": ""
},
"description": "A Great Invoice",
"dueDate": "2024-12-04T00:00:00.000Z",
"issueDate": "2024-11-04T00:00:00.000Z",
"items": [
{
"description": "1st Line Item",
"quantity": "1",
"unitAmount": "2",
"taxRate": "10.0"
},
{
"description": "2nd Line Item",
"quantity": "1",
"unitAmount": "2",
"externalData": [
{
"connectorType": "XERO",
"templateId": "a0208064-d584-4845-b11a-32bca3f5b25a"
}
]
}
]
}'
Explanation:
Body:
templateId
: optional, refers to the XeroItemID
. You can find out more about the XeroItemID
in the Xero documentation.
Example response
{
"id": "d72a4923-ad34-4a52-bb62-55c61474e813",
"invoiceNo": "INV-6666",
"description": "A Great Invoice",
"totalAmount": "4.2",
"taxAmount": "0.2",
"dueAmount": "4.2",
"dueDate": "2024-12-04T00:00:00.000UTC",
"issueDate": "2024-11-04T00:00:00.000UTC",
"creationTime": "2024-11-07T14:57:13.984UTC",
"lastUpdatedTime": "2024-11-07T14:57:13.984UTC",
"status": "DRAFT",
"statusReasonCode": "PENDING_ACTIVATION",
"customer": {
"id": "786aca1d-8286-49c5-8a06-3975bd4657d4",
"name": "Chris Demo Ltd",
"creationTime": "2024-11-07T14:56:23.619UTC",
"people": [
{
"firstName": "Chris",
"lastName": "Carty",
"email": "[email protected]",
"phoneNo": "+44567123478"
}
],
"addresses": [
{
"city": "London",
"postalCode": "1245",
"country": "UK",
"addressLine1": "Street"
}
],
"directDebitMandate": {},
"timezone": "Europe/London"
},
"items": [
{
"description": "1st Line Item",
"quantity": 1,
"unitAmount": 2,
"taxRate": 10,
"taxAmount": 0.2,
"totalAmount": 2.2,
"externalData": []
},
{
"description": "2nd Line Item",
"quantity": 1,
"unitAmount": 2,
"taxAmount": 0,
"totalAmount": 2,
"externalData": [
{
"connectorType": "XERO",
"templateId": "a0208064-d584-4845-b11a-32bca3f5b25a"
}
]
}
],
"paymentRequests": [
{
"description": "A Great Invoice",
"amount": 4.2,
"payByDate": "2024-12-04T00:00:00.000UTC",
"creationTime": "2024-11-07T14:57:13.984UTC",
"lastUpdatedTime": "2024-11-07T14:57:13.984UTC",
"statusReasonCode": "PENDING_ACTIVATION",
"paymentLink": {
"url": "https://app.staging.adfin.com/pay/lt0LJVOFz0zlbnbIeC"
}
}
],
"creditNotes": []
}
Step 3 (option 1): Activate the invoice
Using the id
generated for the invoice in Step 2, you can now activate the invoice.
Activating an invoice will trigger a one-time payment workflow. This workflow includes:
- Invoice will be sent to the customer via email on the
issueDate
. The email will contain a payment link with the call to action 'Pay this invoice!' - Adfin will initiate a schedule of reminders for this invoice.
curl -X PUT "https://api.adfin.com/v1/api/invoices/{invoiceId}:activate" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer your_access_token" \
-d '{
"collectionMethod": "ONE_TIME_PAYMENT",
"customMessage": "Thanks in advance!"
}'
Explanation:
Body:
collectionMethod
: required field, needs to be set toONE_TIME_PAYMENT
.customMessage
: optional field, the message will be included in the invoice email sent to the customer. See an example below 'Thanks in advance!'.
Example Adfin one-time payment email:

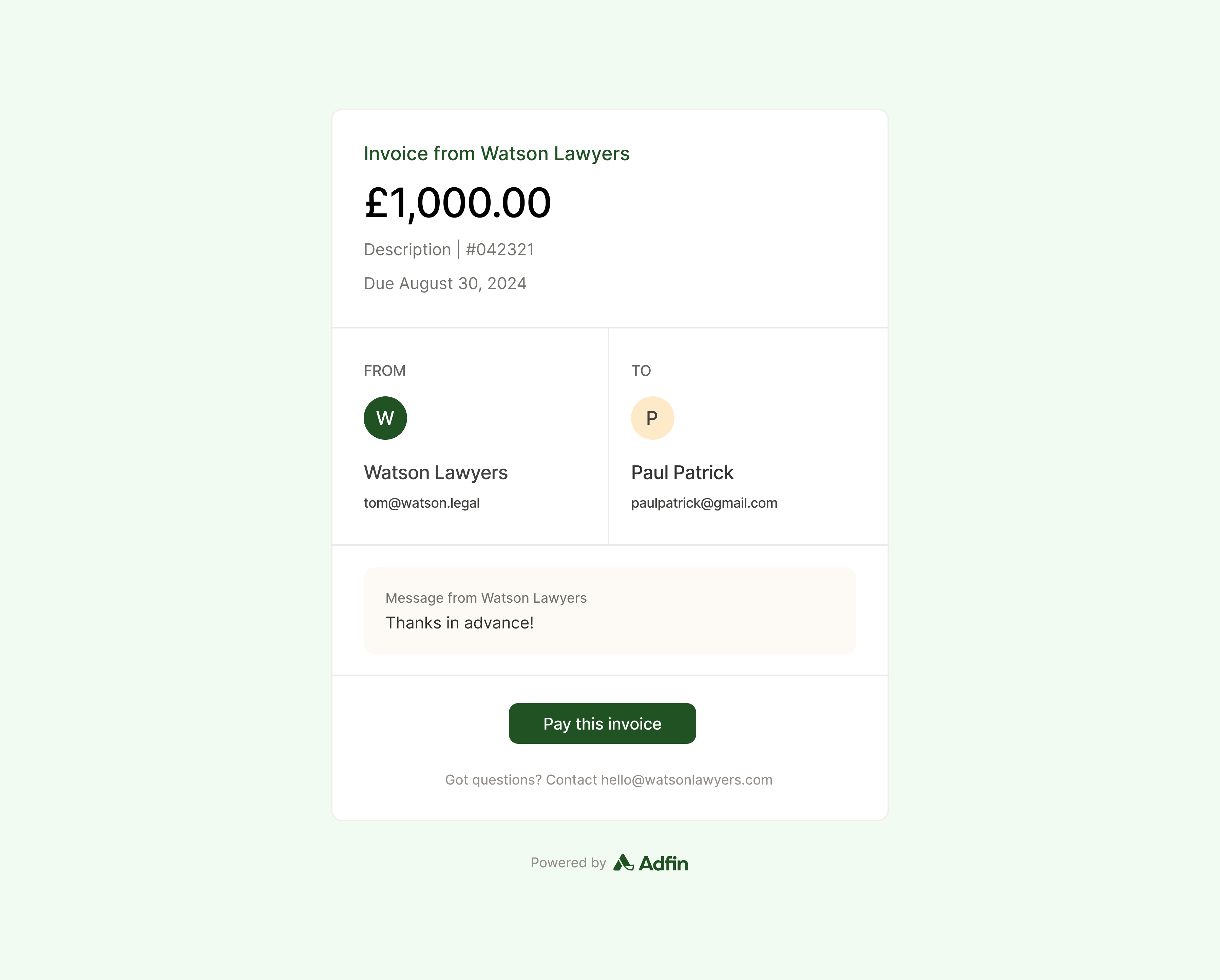
Step 3 (option 2): Redirect customer to one-time payment page
If you’d prefer not to activate a one-time payment workflow and just want to share the payment link directly with the customer, simply use the URL in the url
field (see the example response in Step 2). This will take the customer straight to the one-time payment page, hosted by Adfin!
URL example:
"url": "https://app.staging.adfin.com/pay/lt0LJVOFz0zlbnbIeC"
Example Adfin hosted one-time payment page:
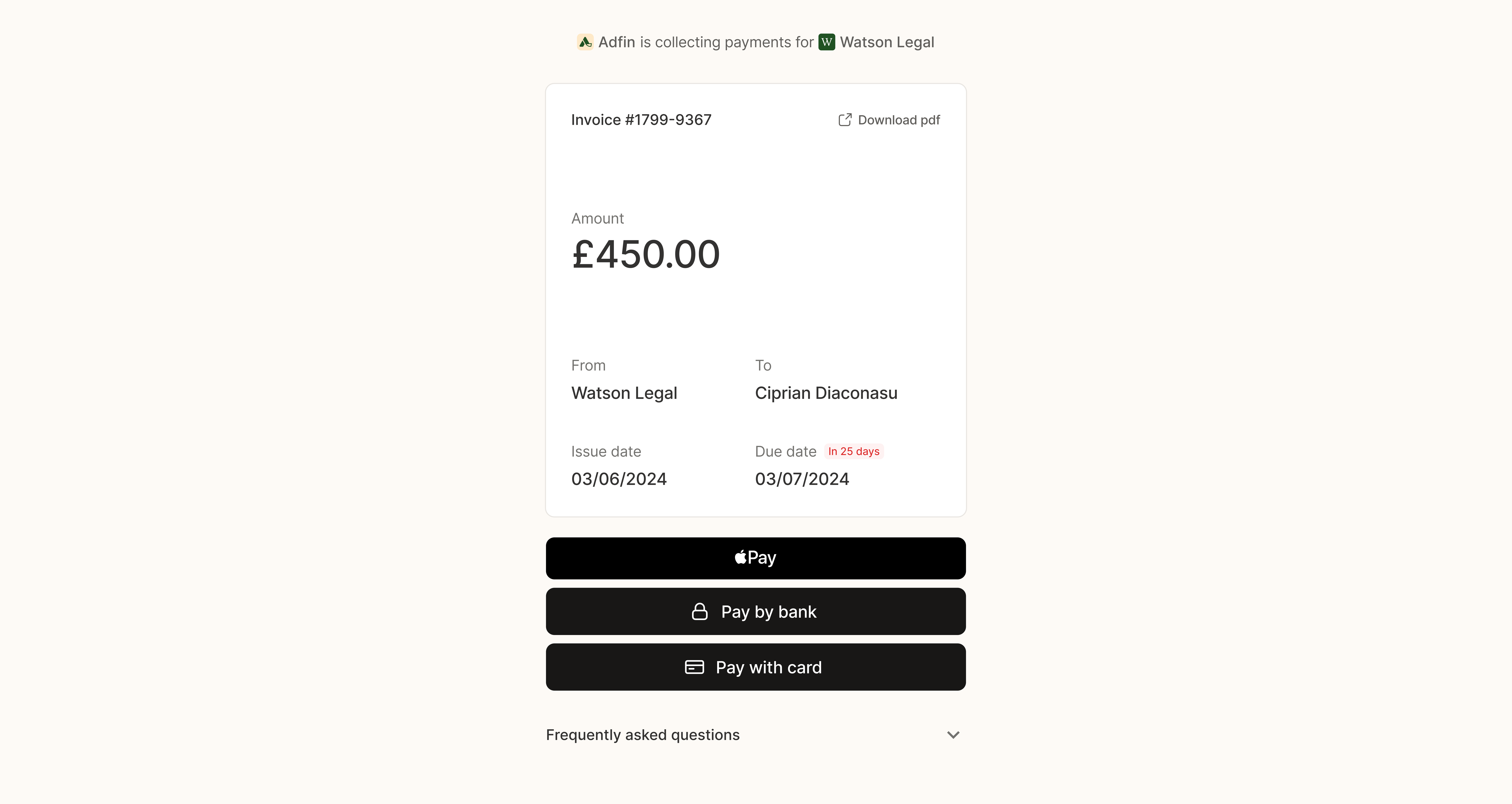
Updated 4 months ago